1.環境
(1)開発環境
OS: Windows 10 Pro
IDE:Eclipse IDE for Enterprise Java Developers Version: 2020-12 (4.18.0)
Java version:11.0.9、Tomcat v8.0
(2)データベース
XAMPPのmysql
mysql Ver 15.1 Distrib 10.4.17-MariaDB, for Win64 (AMD64)
2.アプリケーションの概要
データベースに対して、ユーザー名の登録、参照、更新、削除を行う。
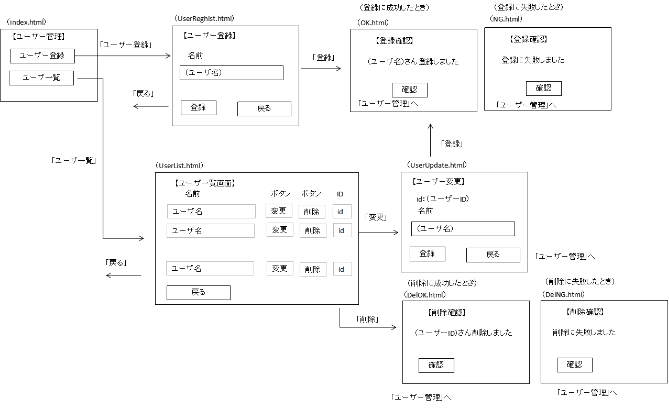
3.設計
(1)CRUD表
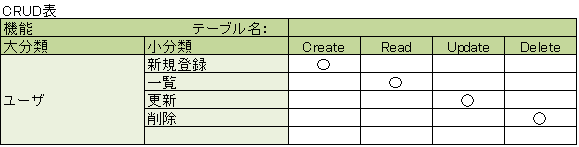
(2)ER図
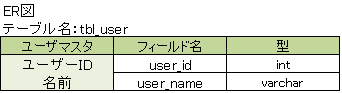
(3)クラス図
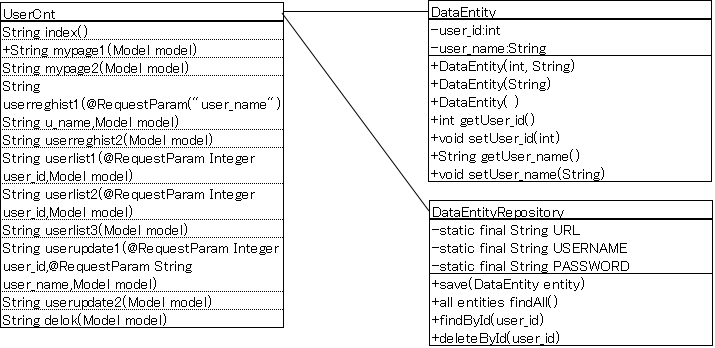
(4)シーケンス図
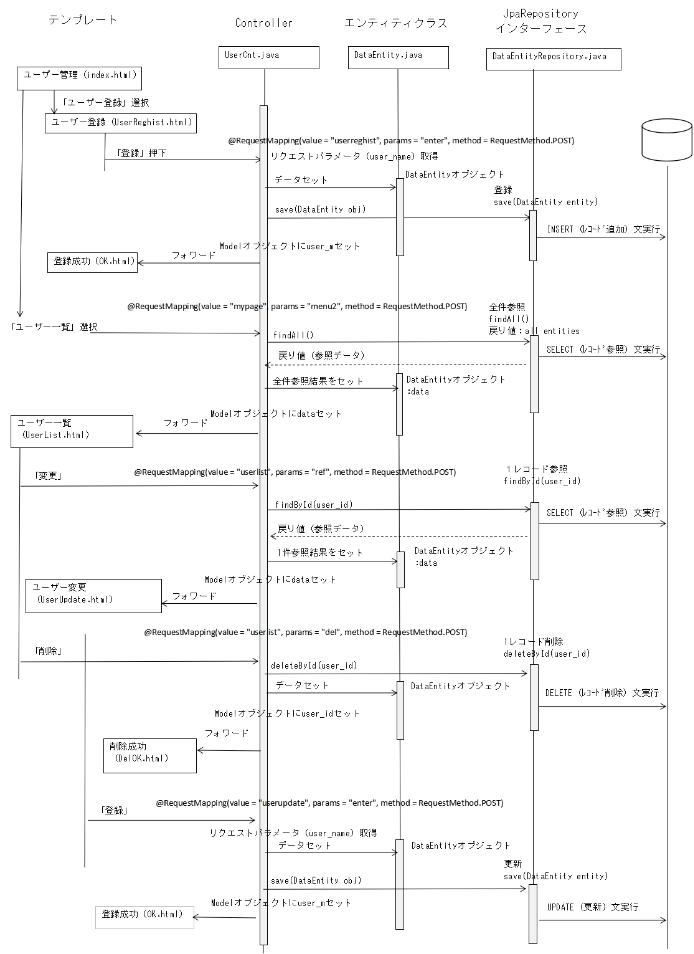
4.実装
(1)プロジェクトフォルダ作成
ファイル>新規>その他を選択し、「ウイザードを選択」画面を表示
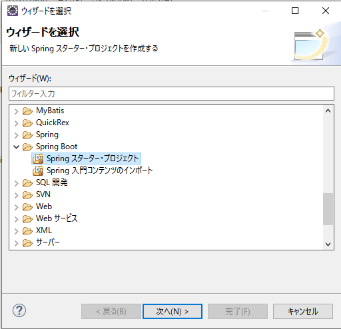
「Springスタータ・プロジェクト」を選択
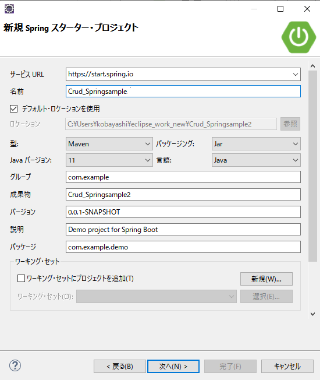
ライブラリの選択
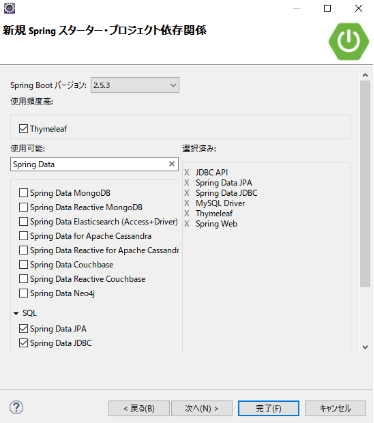
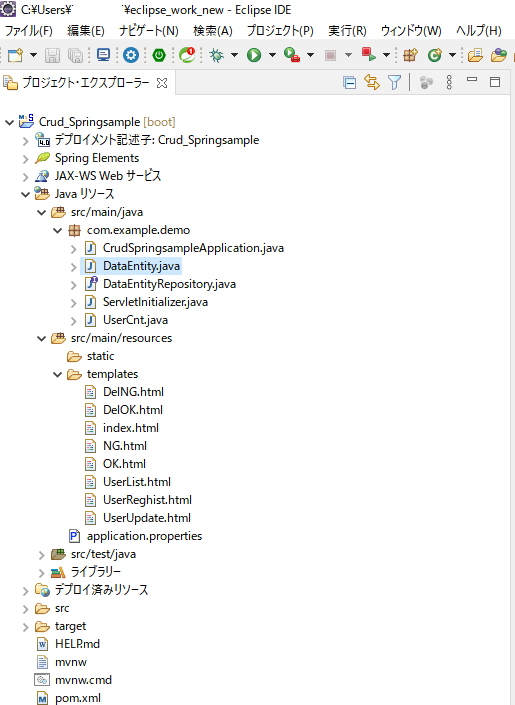
(2)プロパティファイルの編集
Mysqlの接続情報を書く

(3)ソースファイル
テンプレート
index.html(ユーザー管理画面)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
<!DOCTYPE html> <html xmlns:th="http://www.thymeleaf.org"> <head> <meta charset="UTF-8"> <title>メニュー</title> </head> <body> <h2>【ユーザー管理】</h2> <form action="mypage" method="post"> <input type="submit" name="menu1" value="「ユーザー登録」" style="width:200px;height:50px;margin:10px"><br> <input type="submit" name="menu2" value="「ユーザー一覧」" style="width:200px;height:50px;margin:10px"> </form> </body> </html> |
UserReghist.html(ユーザー登録画面)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
<!DOCTYPE html> <html xmlns:th="http://www.thymeleaf.org"> <head> <meta charset="UTF-8"> <title>登録</title> </head> <body> <h2>【ユーザー登録】</h2> <form action="userreghist" method="post"> 名前:<input type="text" name="user_name"><br> <input type="submit" name="enter" value="登録"> <input type="submit" name="back" value="戻る"> </form> </body> </html> |
UserList.html(ユーザー一覧画面)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 |
<!DOCTYPE html> <html xmlns:th="http://www.thymeleaf.org"> <head> <meta charset="UTF-8"> <title>ユーザー一覧画面</title> <style type="text/css"> /* 基本のテーブル定義 */ .t {border:1px solid #000000;border-collapse:collapse;table-layout:fixed;font-size:16px} .t td{border:1px solid #000000;height:16px;} .t th{border:1px solid #000000;font-size:16px} .t th{background-color:#FFBB88;color:#000000;} .t tr:nth-child(odd) td{background-color:#C8C8E8;color:#000000;} .t tr:nth-child(even) td{background-color:#E8E8FF;color:#000000;} .t1{width:550px;} .t2{width:50px} .fixed{position: sticky; top: 0;} #data { overflow-x:scroll;overflow-y:scroll; width:700px;height:245px; } </style> </head> <body> <h2>【ユーザー一覧】</h2> <div id="data"> <table class=t> <tr> <th class="t1 fixed">名前</th> <th class="t2 fixed">ボタン</th> <th class="t2 fixed">ボタン</th> <th class="t2 fixed">ID</th> </tr> <tr th:each="entity : ${data}"> <td class="t1" th:text="${entity.user_name}"></td> <td> <form th:action="userlist" name="ref" method="post" > <input type="submit" name="ref" class="t2" value="変更"> <input type="hidden" name="user_id" th:value="${entity.user_id}"> </form> </td> <td> <form th:action="userlist" method="post" > <input type="submit" name="del" class="t2" value="削除"> <input type="hidden" name="user_id" th:value="${entity.user_id}"> </form> </td> <td class="t2" th:text="${entity.user_id}"></td> </tr> </table> </div> <form th:action="userlist" method="post" > <input type="submit" name="back" value="戻る"> </form> </body> </html> |
UserUpdate.html(ユーザー変更画面)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
<!DOCTYPE html> <html xmlns:th="http://www.thymeleaf.org"> <head> <meta charset="UTF-8"> <title>ユーザー変更</title> </head> <body> <h2>【ユーザー変更】</h2> <form th:action="userupdate" th:object="${data}" method="post"> 名前:<input type="text" name="user_name" th:field="*{user_name}"> <input type="hidden" name="user_id" th:field="*{user_id}"><br> <input type="submit" name="enter" value="登録"> <input type="submit" name="back" value="戻る"> </form> </body> </html> |
OK.html(登録成功確認画面)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
<!DOCTYPE html> <html xmlns:th="http://www.thymeleaf.org"> <head> <meta charset="UTF-8"> <title>登録確認画面</title> </head> <body> <h2>【登録確認】</h2> <p th:text="${user_name}"/>さん登録しました <form action="userreghist" method="post"> <input type="submit" name="back" value="確認" style="width:200px;height:50px;margin:10px"><br> </form> </body> </html> |
DelOK.html(削除成功確認画面)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
<!DOCTYPE html> <html xmlns:th="http://www.thymeleaf.org"> <head> <meta charset="UTF-8"> <title>削除確認画面</title> </head> <body> <h2>【削除確認】</h2> <p th:text="${user_id}"/>さん削除しました<br> <form action="delok" method="post"> <input type="submit" name="back" value="確認" style="width:200px;height:50px;margin:10px"><br> </form> </body> </html> |
UserCnt.java(コントローラ)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 |
package com.example.demo; import java.util.List; import java.util.Optional; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.RequestParam; @Controller public class UserCnt { @Autowired DataEntityRepository repository; @RequestMapping(value = "/", method = RequestMethod.GET) public String index() { return "index"; } @RequestMapping(value = "mypage", params = "menu1", method = RequestMethod.POST) public String mypage1(Model model) { return "UserReghist"; } @RequestMapping(value = "mypage", params = "menu2", method = RequestMethod.POST) public String mypage2(Model model) { //データベースから一覧取得して、オブジェクトに入れる List<DataEntity> data = repository.findAll(); //テンプレートで表示する値をセット model.addAttribute("data",data); return "UserList"; } @RequestMapping(value = "userreghist", params = "enter", method = RequestMethod.POST) public String userreghist1( @RequestParam("user_name")String u_name, Model model) { //テンプレートで表示する値をセット model.addAttribute("user_name", u_name); //DBに1レコード保存する repository.save(new DataEntity(u_name)); return "OK"; } @RequestMapping(value = "userreghist", params = "back", method = RequestMethod.POST) public String userreghist2(Model model) { return "index"; } @RequestMapping(value = "userlist", params = "ref", method = RequestMethod.POST) public String userlist1( @RequestParam Integer user_id, Model model) { //データベースから検索取得して、オブジェクトに入れる Optional<DataEntity> data = repository.findById(user_id); //テンプレートで表示する値をセット model.addAttribute("data",data); return "UserUpdate"; } @RequestMapping(value = "userlist", params = "del", method = RequestMethod.POST) public String userlist2(@RequestParam Integer user_id, Model model) { //テンプレートで表示する値をセット model.addAttribute("user_id", user_id); //DBから削除する repository.deleteById(user_id); return "DelOK"; } @RequestMapping(value = "userlist", params = "back", method = RequestMethod.POST) public String userlist3(Model model) { return "index"; } @RequestMapping(value = "userupdate", params = "enter", method = RequestMethod.POST) public String userupdate1(@RequestParam Integer user_id, @RequestParam String user_name, Model model) { //テンプレートで表示する値をセット model.addAttribute("user_name", user_name); repository.save(new DataEntity(user_id,user_name)); return "OK"; } @RequestMapping(value = "userupdate", params = "back", method = RequestMethod.POST) public String userupdate2(Model model) { return "index"; } @RequestMapping(value = "delok", params = "back", method = RequestMethod.POST) public String delok(Model model) { return "index"; } } |
使用したアノテーション
@Controller
コントローラとして認識され、returnで指定したテンプレートをレンダリングして表示する
@Autowired
インスタンスを生成する
@RequestMapping
マッピングするアドレスの指定とGET/POSTメソッドの種類を指定
@RequestParam
送信される項目の名前をもつパラーメータ値を割り当てる
DataEntity.java(エンティティクラス)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 |
package com.example.demo; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.Id; @Entity public class DataEntity { @Id @GeneratedValue private Integer user_id; private String user_name; public DataEntity() { super(); } public DataEntity(Integer user_id) { super(); this.user_id = user_id; } public DataEntity(String user_name) { super(); this.user_name = user_name; } public DataEntity(Integer user_id, String user_name) { super(); this.user_id = user_id; this.user_name = user_name; } public Integer getUser_id() { return user_id; } public void setUser_id(Integer user_id) { this.user_id = user_id; } public String getUser_name() { return user_name; } public void setUser_name(String user_name) { this.user_name = user_name; } @Override public String toString() { return "DataEntity [user_id=" + user_id + ", user_name=" + user_name + "]"; } } |
使用したアノテーション
@Entity
エンティティクラスとして認識される
@Id
プライマリキーを指定
@GeneratedValue
自動的に値が生成される
1 2 3 4 5 6 7 8 |
package com.example.demo; import org.springframework.data.jpa.repository.JpaRepository; public interface DataEntityRepository extends JpaRepository<DataEntity, Integer> { } |
DataEntityRepository.java(JpaRepositoryインターフェース)
1 2 3 4 5 6 7 8 |
package com.example.demo; import org.springframework.data.jpa.repository.JpaRepository; public interface DataEntityRepository extends JpaRepository<DataEntity, Integer> { } |
pom.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 |
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.5.3</version> <relativePath/> <!-- lookup parent from repository --> </parent> <groupId>com.example</groupId> <artifactId>Crud_Springsample</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>war</packaging> <name>Crud_Springsample</name> <description>Demo project for Spring Boot</description> <properties> <java.version>11</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jdbc</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-jdbc</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <scope>runtime</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-tomcat</artifactId> <scope>provided</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project> |
(4)データベースとテーブル作成
データベースは自動的に作成される
データベース名:crud_sample
テーブル名:data_entity(フィールド値を保存)
hibernate_sequence(シーケンス番号を保存)
5.実行
(1)XAMPPのMySQLサーバを起動する
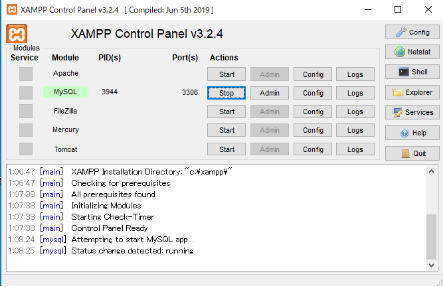
(2)アプリケーションを選択して、実行ボタン>「SpringBootアプリケーション」を押す
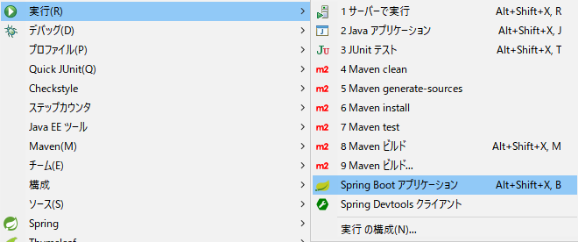
(3)ブラウザからWebサーバに接続
http://localhost:8080/
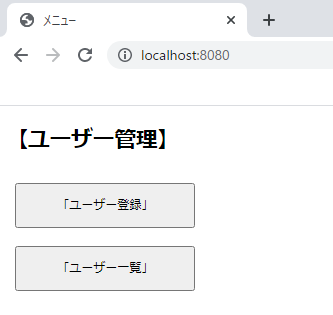
「ユーザー登録」ボタン押下
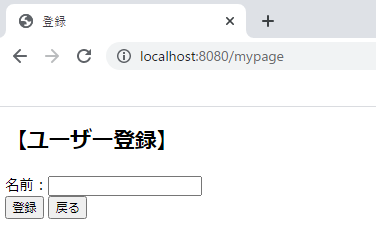
名前:テストネームを入力し、「登録」ボタン押下
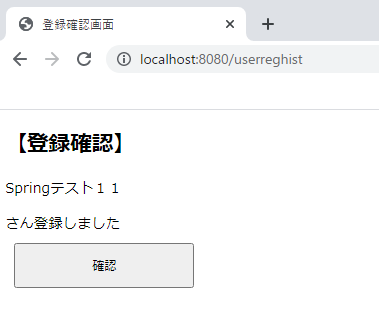
「ユーザー管理」画面で「ユーザー一覧」ボタン押下
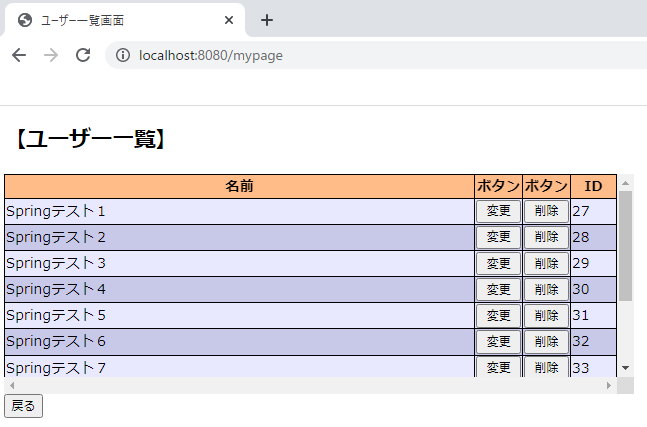
「変更」ボタン押下
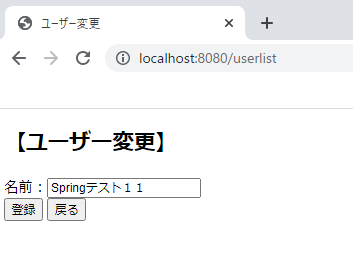
「削除」ボタン押下
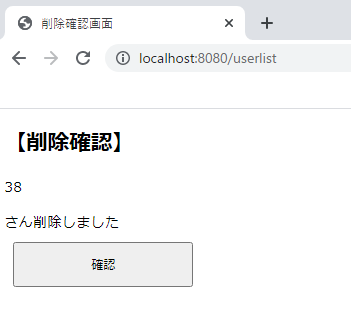
The subject ends herewith.