1.配列とは
配列は、同じデータ型の要素を番号(添字:インデックス)順に並べたものを表します。
添字は、C言語を含む多くの言語は0スタートとなります。
(例)要素数100のときの1次元配列a、a[0]・・・a[99]
配列のサンプル(1)
list_sample1.c
#include <stdio.h>
#define MAX_SIZE 100
int list[MAX_SIZE];//list[0]...list[99]
int size = 0;
void insert(int value) {
if(size < MAX_SIZE) {
list[size++] = value;
printf("list[%d]:%d\n ", size-1,list[size-1]);
}
}
void remov(int index) {
if(index < size) {
for(int i=index; i<size-1; i++) {
list[i] = list[i+1];
}
size--;
}
}
int get(int index) {
if(index < size) {
return list[index];
}
return -1; // return -1 to indicate an error or invalid index
}
int search(int value) {
for(int i=0; i<size; i++) {
if(list[i] == value) {
return i;
}
}
return -1; // return -1 to indicate not found
}
int main(void){
insert(10);//list[0]:10
insert(20);//list[1]:20
insert(30);//list[2]:30
printf("%d\n ", get(1));//20
printf("%d\n ", search(20));//1
remov(1);//list[0]:10,list[1]:30
printf("%d\n ", get(0));//10
printf("%d\n ", get(1));//30
printf("%d\n ", get(2));//-1
return 0;
}
例では、配列の要素数MAX_SIZE変数を定義します。insert関数はリストの末尾に新しい要素を挿入し、remov関数は特定のインデックスの要素を削除します。get関数は特定のインデックスの要素を返し、search関数は特定の値を持つ要素を検索してそのインデックスを返します。
ここでは要素はint型としていますが、他のデータ型の要素を格納する必要がある場合は、配列listのデータ型と関数のパラメーターと戻り値を変更する必要があります。
実行結果
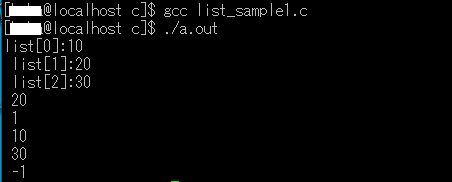
配列のサンプル(2)
list_sample2.c
#include <stdio.h>
#define MAX_SIZE 100 // maximum size of the list
struct List {
int items[MAX_SIZE];
int size;
};
// initialize the list
void initList(struct List *list) {
list->size = 0;
}
// add an item to the list
void addItem(struct List *list, int item) {
if (list->size == MAX_SIZE) {
printf("List is full\n");
return;
}
list->items[list->size++] = item;
}
// remove an item from the list
void removeItem(struct List *list, int index) {
if (index < 0 || index >= list->size) {
printf("Invalid index\n");
return;
}
for (int i = index; i < list->size - 1; i++) {
list->items[i] = list->items[i+1];
}
list->size--;
}
// get an item from the list
int getItem(struct List *list, int index) {
if (index < 0 || index >= list->size) {
printf("Invalid index\n");
return -1;
}
return list->items[index];
}
// print the list
void printList(struct List *list) {
for (int i = 0; i < list->size; i++) {
printf("%d ", list->items[i]);
}
printf("\n");
}
int main(void){
struct List list;
initList(&list);
addItem(&list, 10);
addItem(&list, 20);
addItem(&list, 30);
printList(&list); // prints "10 20 30\n"
removeItem(&list, 1);
printList(&list); // prints "10 30\n"
printf("%d\n", getItem(&list, 1)); // prints "30\n"
return 0;
}
この例では構造体を作成して、構造体を呼び出して配列を操作します。
実行結果
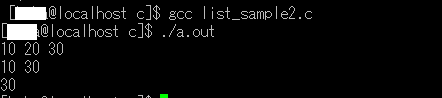
2.線形リスト
線形リストは、データと「次のデータを指し示すポインタ(場所)」が入ったノードと呼ばれる要素がポインタでつながっているデータ構造で、隣接するデータ同士をポインタで連結して表現します。

線形リストのサンプル
list_sample3.c
#include <stdio.h>
#include <stdlib.h>
//ノード
struct Node {
int data;
struct Node *next;
};
struct Node *head = NULL;
void insert(int value) {
struct Node *newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = value;
newNode->next = NULL;
if(head == NULL) {
head = newNode;
} else {
struct Node *current = head; //headのノードから最後のノードを順番に調べて
while(current->next != NULL) { //その次に新しいノードを追加する
current = current->next;
}
current->next = newNode;
}
}
void display() {
if(head == NULL) {
printf("List is empty.\n");
} else {
struct Node *current = head;
while(current != NULL) { //次のノードがNULLポインタになるまで繰り返す
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
}
int main() {
insert(5);
insert(10);
insert(15);
display();//5 10 15
return 0;
}
データとリスト内の次のノードへのポインターを保持するために、Nodeと呼ばれる構造体を定義します。関数insertはリストの最後に新しいノードを追加し、関数displayは各ノードのデータを出力します。
main関数では、3 つの値を挿入し、結果のリストを表示します。
実行結果

end